tell application "applicationname"
end tell
The first line of the script tells the application "applicationname" to do what is between the tell and the end tell part. End tell, tells the script editor to stop telling that application to do commands. Whenever you use a tell command you must also have an end tell command.
Here is an example script:
tell application "iTunes" activate end tell
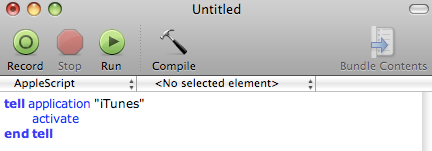
This tells the application "iTunes" to do the command(s) until the end tell. The command(s) in this script is activate, which activates the application iTunes. Another script is this:
tell application "iTunes" quit end tell
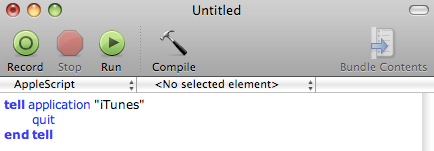
This tells the application "iTunes to quit. To find an applications name and the commands you can use with them, go to the Script Editor -> File -> Open Dictionary and then select the application you would like to use apple script to control. Lets for example look at iTunes. Scan through the commands and we see the command next track. The v after next track can be thought of as verb, meaning it is an action.

Lets make a script out of that command. The script will look like this:
tell application "iTunes" next track end tell
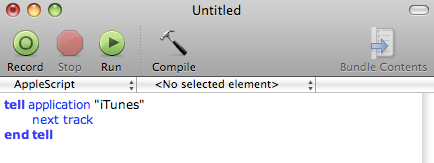
Running this script will make itunes play the next track. Lets look at another command, play.

Play is an action command as stated by the v after play. The words after : are the description of those commands. Anything within the bracket [information] is optional. We can make a script that just uses play:
tell application "iTunes" play end tell
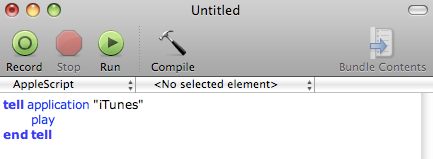
This will tell iTunes to play. If iTunes is on pause and you run this script iTunes will play. If we look back to our play command there was an optional [specifier] : item to play. We can have an item after the play command and it will play that item. Here is an item, track.

Track can be thought of as a noun because of the n after it. This means it isn't an action just a person, place, or thing. Now since we know our commands we can make a neat script:
First we start out with what we learned in our last section and type:
display dialog "What song would you like to play?" default answer ""
set play_song to text returned of result
Remember this displays a box with the text "What song would you like to play?" and lets you type in an answer. Then it sets the play_song variable to what was typed in.
Now we can use that with what we learned in this section and add to the script.
display dialog "What song would you like to play" default answer "" set play_song to text returned of result tell application "iTunes" try play track play_song end try end tell
- We know what the first two lines mean.
- The third line tells the application "iTunes" do to the commands until the end try command
- The next line says try. Try is another command like tell. Try, tries to do everything between it and the end try command. I will explain why we use the try command later
- The next line with play the track play_song which is a variable that stands for whatever the user types to answer the question "What song would you like to play?"
- The last two lines end the try command and end the tell command
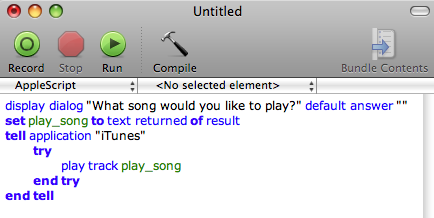
Run the script and type in a song name. If the song name is in your iTunes it will play it. The reason we used the try command is because if we didn't and we typed in a song name that was in our iTunes library we would get this error:
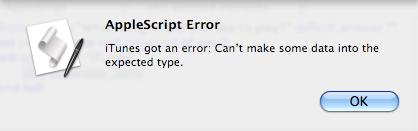
This is because we told iTunes to play a song that isn't there so it errors. When we use the try command to play a song that isn't there it will try and if it errors it won't stop the script, but it will end the try command.